In a crypto, stock, or foreign exchange, speed and efficiency play an important role, especially for matching engines that process buy and sell orders. A ring buffer is a circular data structure that can improve the performance of the exchange by minimizing memory overhead and ensuring consistent data flow. This article will provide you with a comprehensive guide to developing a matching engine using a ring buffer.
Matching engine (ME - Order Matching System)
1. Definition
2. How it works
- Receiving order: The matching engine will receive buy or sell orders from users with information such as symbol, price, quantity, and order type (market order, limit order…).
- Order Storage: Orders are stored in the Order book, which consists of two main lists:
- Buy Orders: A list of buy orders sorted in descending price order.
- Sell Orders: A list of sell orders sorted in ascending price order.
- Order Matching: The matching engine checks if buy and sell orders can be matched. Then, the orders will be matched following the common order matching rules :
- Price: A buy order can match with a sell order if the buy price is higher than or equal to the selling price.
- Time: If multiple orders are at the same price, the order placed earlier will be prioritized.
- Trade Execution: If a buy order and a sell order match, the transaction will be executed. The quantity of stock is transferred from the seller to the buyer, and the money is transferred from the buyer to the seller.
- Updating the order book: After the trade is executed, the order book is updated to reflect matched orders and the remaining amount of the orders.
For example, we have two orders:
- Buy order: 5 ETH for $100
- Sell order: 2 ETH for $99
When a sell order for 2 ETH at $99 is submitted, the matching engine will match this order with the first buy order in the order book. As a result, a transaction of 2 ETH at $99 will be executed. Once the trade is completed, the matching engine will store the remaining buy order amount in the buy order queue, or vice versa.
3. Common types of orders
- Market Order: A buy or sell order is at the current best price.
- Limit Order: A buy or sell order is at the specified price or better.
- Stop Order: An order when the price reaches the specified price.
- Stop-Limit Order: An order can be turned into a limit order when the price reaches a specific level.
Ring Buffer Overview
1. Definition
- Fixed Size: The Ring Buffer has a fixed size, so when it becomes full, the new element will overwrite the oldest element.
- Two Pointers: Head Pointer points to the most recent position, and Tail Pointer points to the oldest position.
- Efficient Memory Usage: The Ring Buffer does not need to move data when writing or reading, which optimizes memory usage and improves performance.
```bash
npm install react-native-maps or yarn add react-native-maps
```
2. Typical use cases
- Audio or video processing.
- Inter-process communication.
- Queues in real-time systems
<application>
<meta-data
android:name="com.google.android.geo.API_KEY"
android:value="YOUR_GOOGLE_MAPS_API_KEY" />
</application>
3. Benefits
- High performance due to no need for data shifting when adding or removing elements.
- Efficient memory usage with a fixed size.
4. Drawbacks
- Fixed size can be limited if the amount of data changes unpredictably.
- More complex in managing full and empty states
5. How it works
- Implemented on an array, using two pointers: tail and head.
- When a write command is received, the head pointer writes data to the buffer and then increments by 1.
- When a read command is received, data is read from the buffer, and the tail is incremented by 1.
- When a pointer reaches the last element, it automatically points back to the initial position.
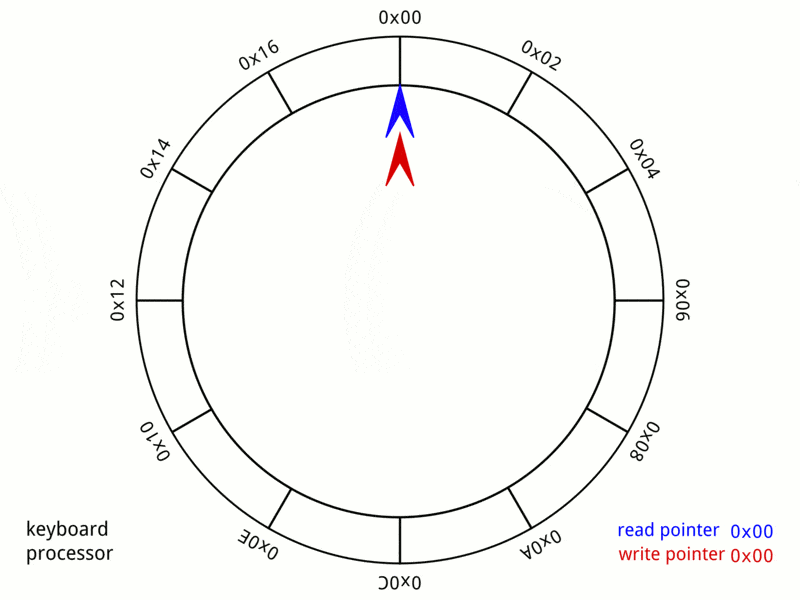
Here is the command
// Does not allow overwriting
IF (TAIL + 1) === SIZE ( throw IS_FULL), TAIL = (TAIL + 1) % SIZE
For example:
SIZE = 5 We have:
Buffer: [ , , , , ]
HEAD = 0
TAIL = 0
- Open ios/Podfile and add the following snippets:
// Plus 1,
Buffer: [1, , , , ]
HEAD = 0
TAIL = 1
// Plus 2.
Buffer: [1, 2, , , ]
HEAD = 0
TAIL = 2
// Plus 3.
Buffer: [1, 2, 3, , ]
HEAD = 0
TAIL = 3
// Plus 4.
Buffer: [1, 2, 3, 4, ]
HEAD = 0
TAIL = 4
// Plus 5.
Buffer: [1, 2, 3, 4, 5]
HEAD = 0
TAIL = 5
// Plus 6.
Throw IS_FULL
// Pop
1,2,3,4,5
// Allows overwriting
IF (TAIL + 1) === SIZE HEAD = (HEAD + 1) % SIZE , TAIL = (TAIL + 1) % SIZE
// Plus 1.
Buffer: [1, , , , ]
HEAD = 0
TAIL = 1
// Plus 2.
Buffer: [1, 2, , , ]
HEAD = 0
TAIL = 2
// Plus 3.
Buffer: [1, 2, 3, , ]
HEAD = 0
TAIL = 3
// Plus 4.
Buffer: [1, 2, 3, 4, ]
HEAD = 0
TAIL = 4
// Plus 5.
Buffer: [1, 2, 3, 4, 5]
HEAD = 0
TAIL = 5
// Plus 6.
Buffer: [6, 2, 3, 4, 5]
HEAD = 0
TAIL = 1
// Plus 6.
Buffer: [6, 7, 3, 4, 5]
HEAD = 0
TAIL = 2
// POP HEAD start = 2
3,4,5,6,7
when you apply a Ring Buffer in the matching engine, it will help optimize the processing of buy and sell orders quickly and efficiently. The Ring Buffer ensures that orders are processed in FIFO order, while the order book and matching algorithm ensure that orders are matched accurately according to predefined rules.
Demo: Application of Machine Engine and Ring Buffer in P2P trading.
In the current financial trading landscape, P2P (peer-to-peer) trading systems are gaining popularity for their transparency and efficiency. To improve the performance and reliability of these systems, it is crucial to implement advanced technologies like the Matching Engine (ME) and Ring Buffer.
Technology used
Database: MYSQL
Backend: Java Spring boot
Front end: NextJs
Workflow
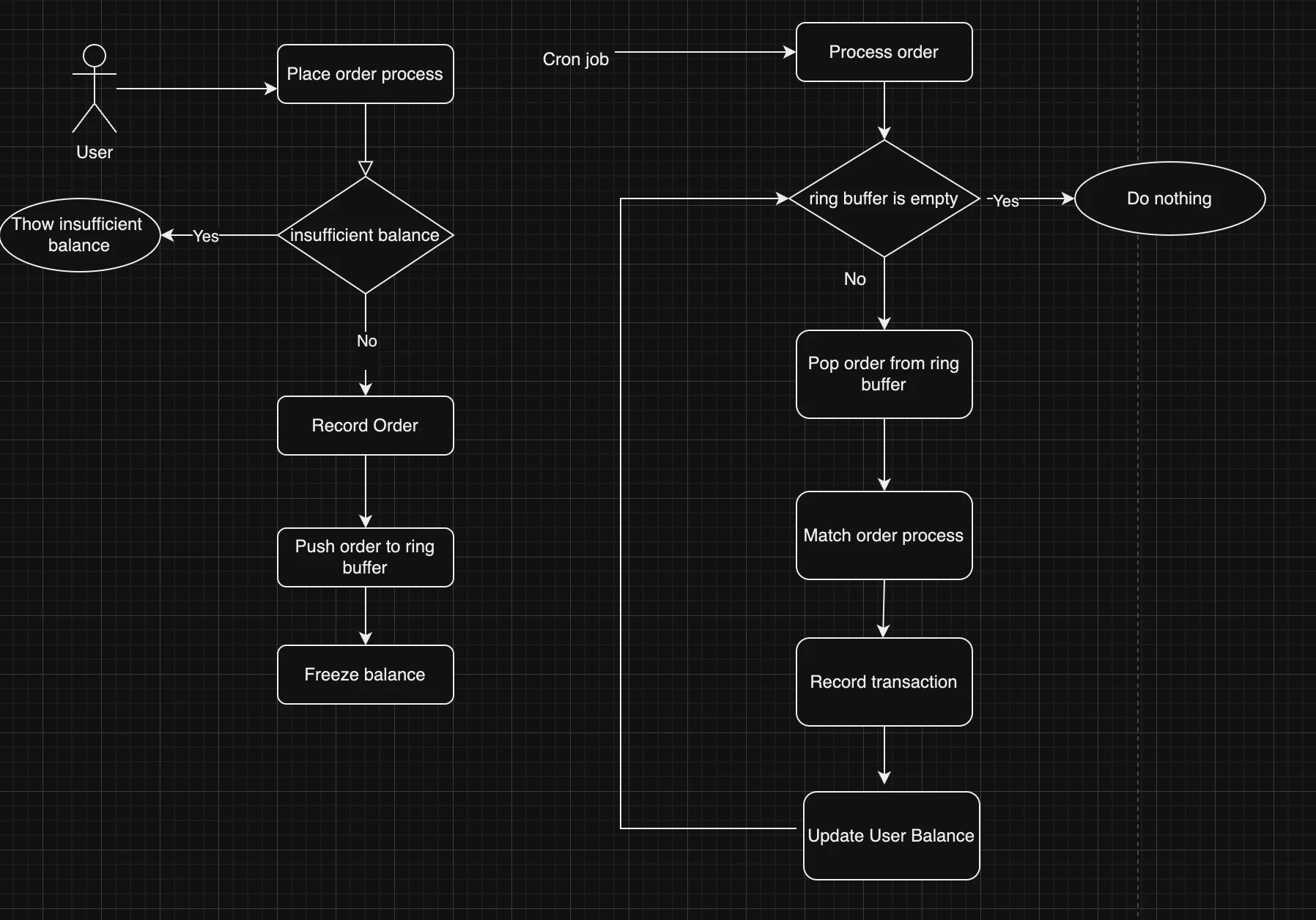
Data Diagram
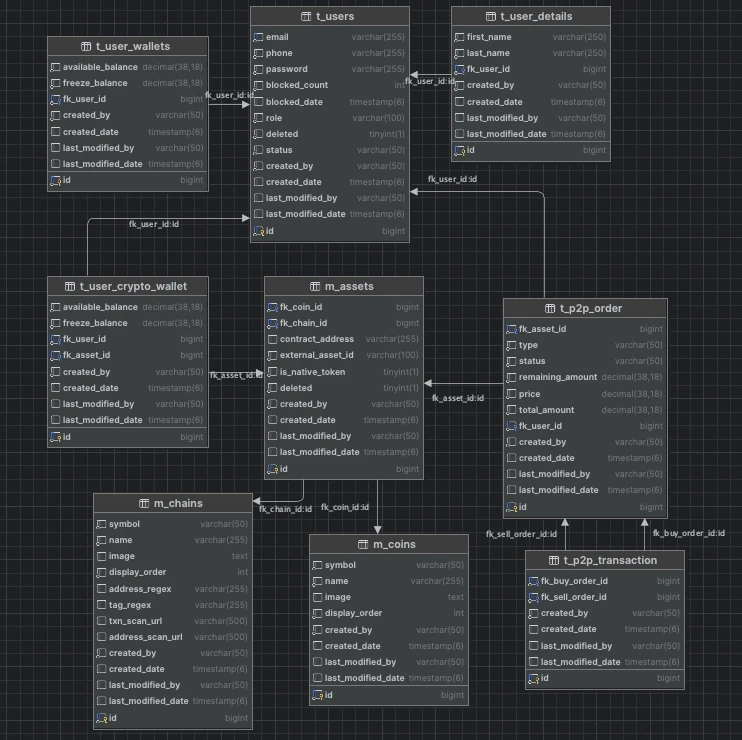
Below is an example of user input
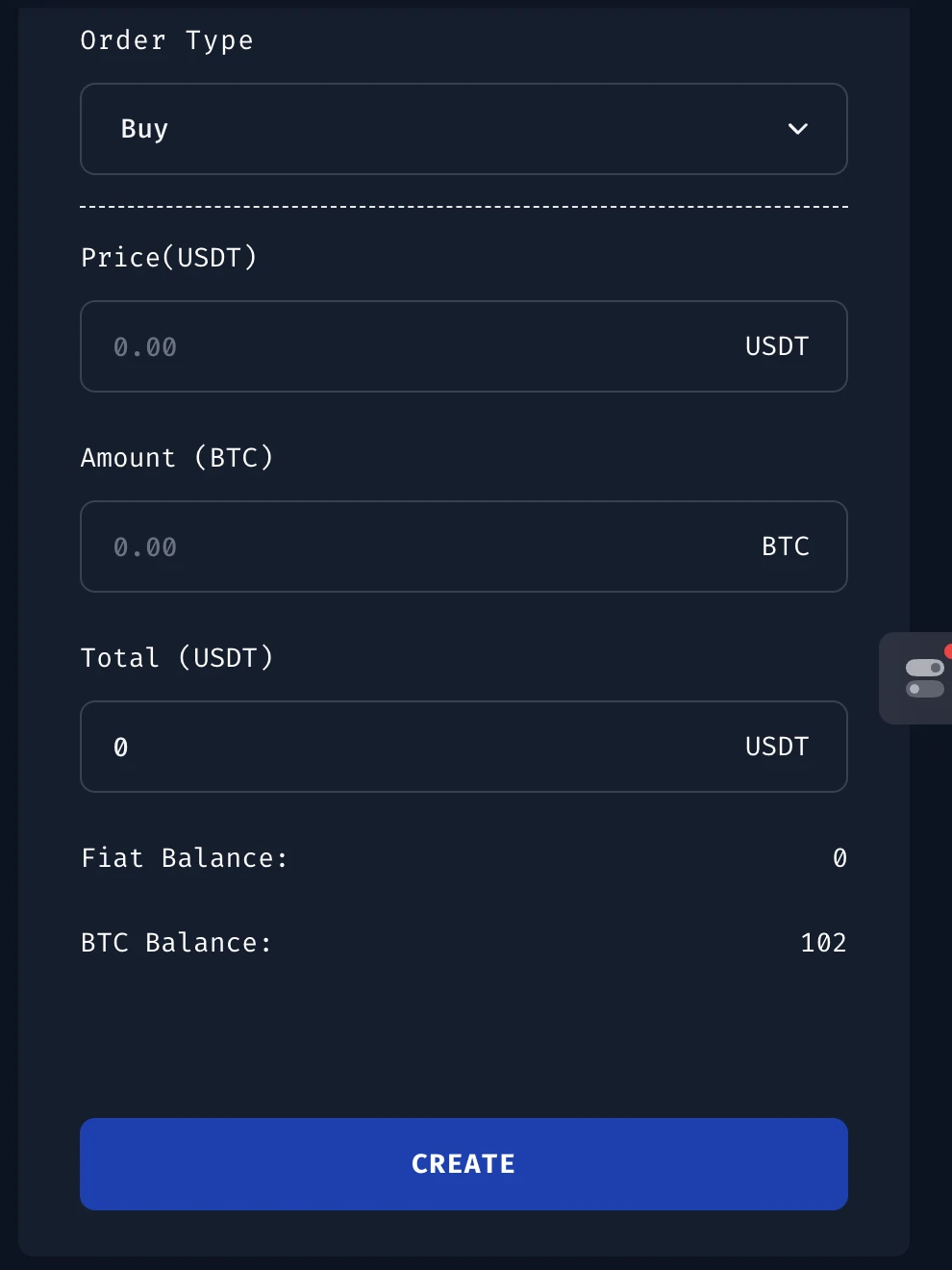
Order type: Select the order type you want.
Price: Enter the price that you want.
Amount: Specify the value of tokens you wish to buy or sell.
Total: The system will compute the total USD needed.
Main Processing Classes
- P2POrderService
- MatchingEngineService
- P2PTransactionService
- UserWalletService
- RingBuffer
- UserCryptoWalletService
P2POrderService
Receives orders and places them into the ring buffer to await processing by the matching engine. It calls the UserWalletService and UserCryptoWalletService to update the user’s balance.
If the transaction type is BUY, the system will freeze the total USD needed in the user’s wallet.


MatchingEngineService
Sequentially retrieve orders from the ring buffer in FCFS order and match them with previous orders to execute P2P transactions.
The transactions are compared to meet the following conditions:
* Buy order: Buy price >= Sell price
* Sell order: Sell price <= Buy price
* Cannot buy from oneself
* Tokens must be the same
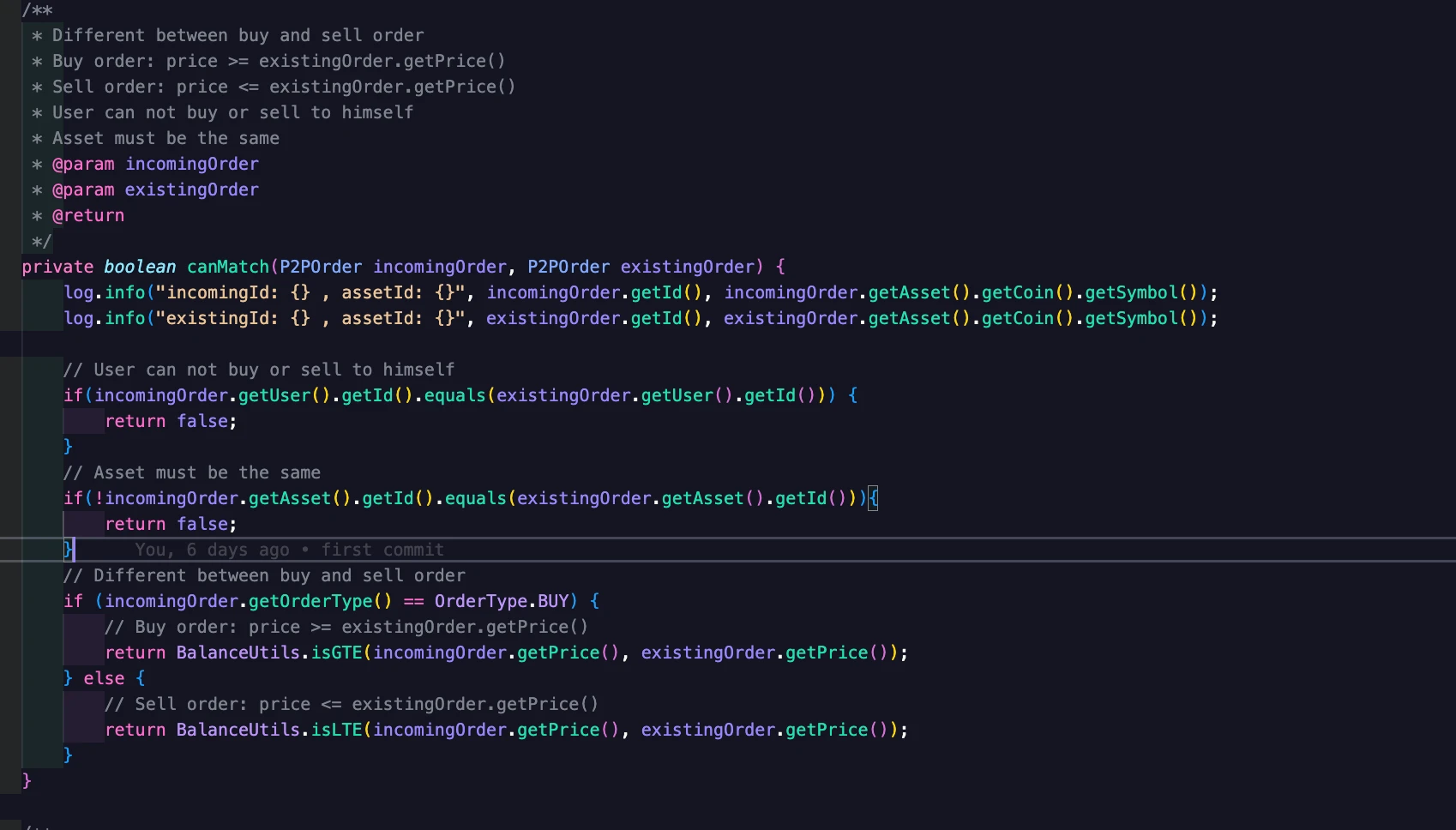
You can use @PostConstruct to declare a single ring buffer for the application with the buffer size specified in the application file.
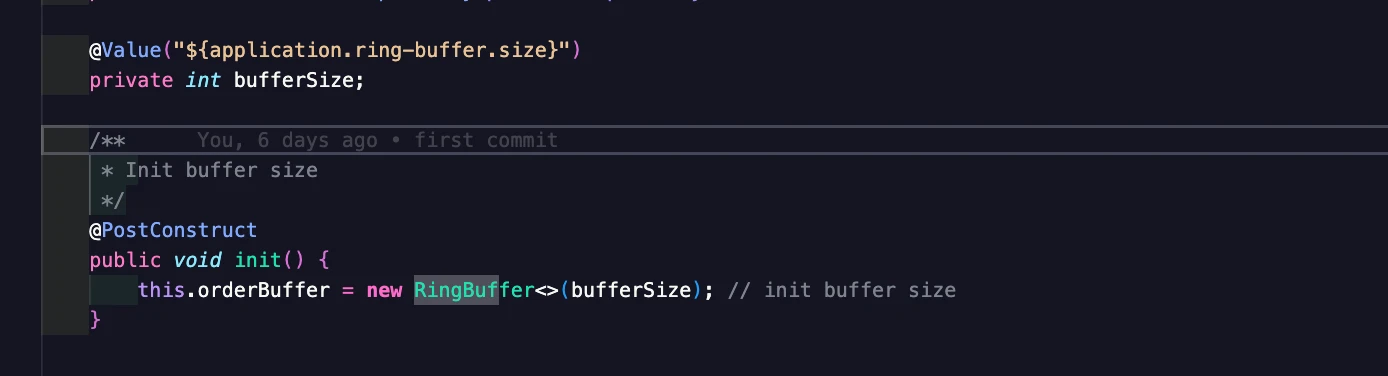
The cron job will call the processOrders function every minute,

@Scheduled(fixedRate = {milisecond})
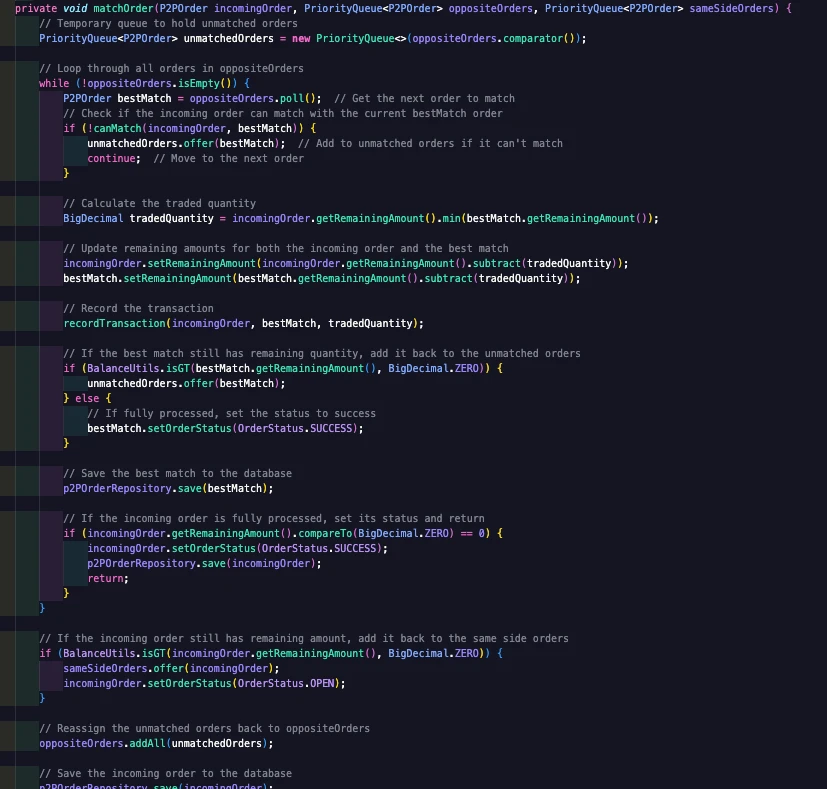
Upon successful order matching, the MatchingEngineService will call the P2PTransactionService to save the transaction history in the database (buyOrder, sellOrder, quantity, price).
Declare Ring buffer
Class RingBuffer includes: size, head, tail, isFull
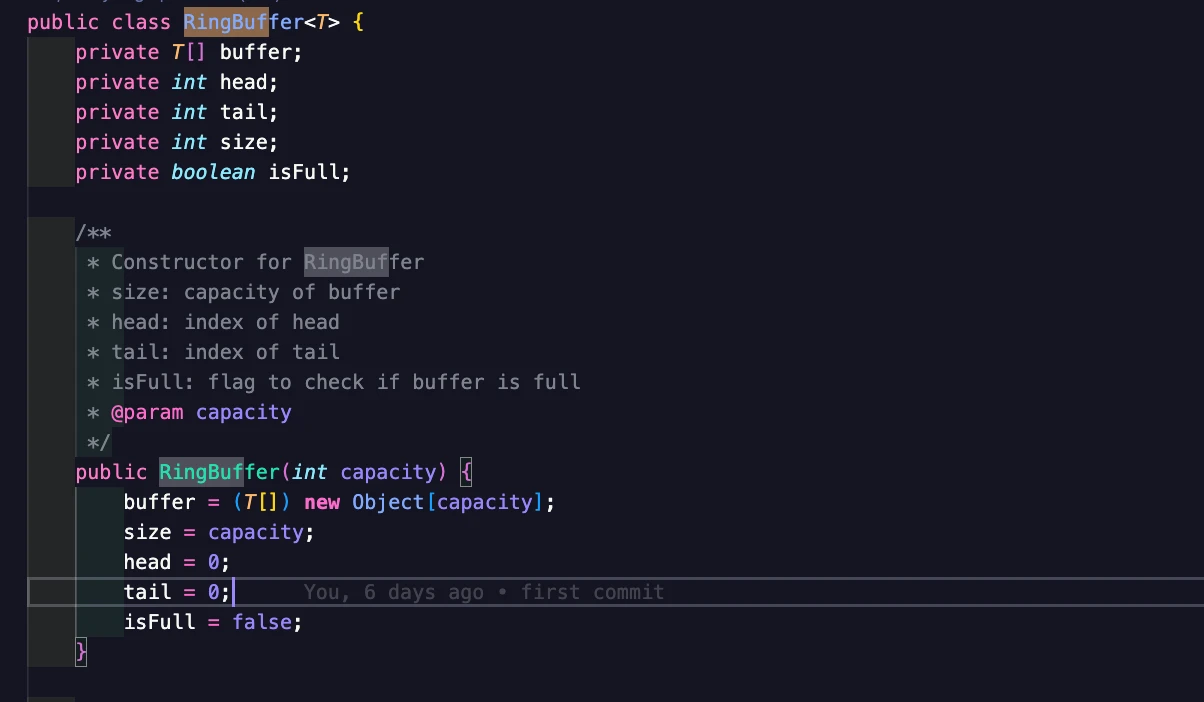
By applying the ring buffer algorithm, we can declare a push function to add a new item to the buffer.
// Does not allow overwriting
IF (TAIL + 1) === SIZE ( throw IS_FULL), TAIL = (TAIL + 1) % SIZE
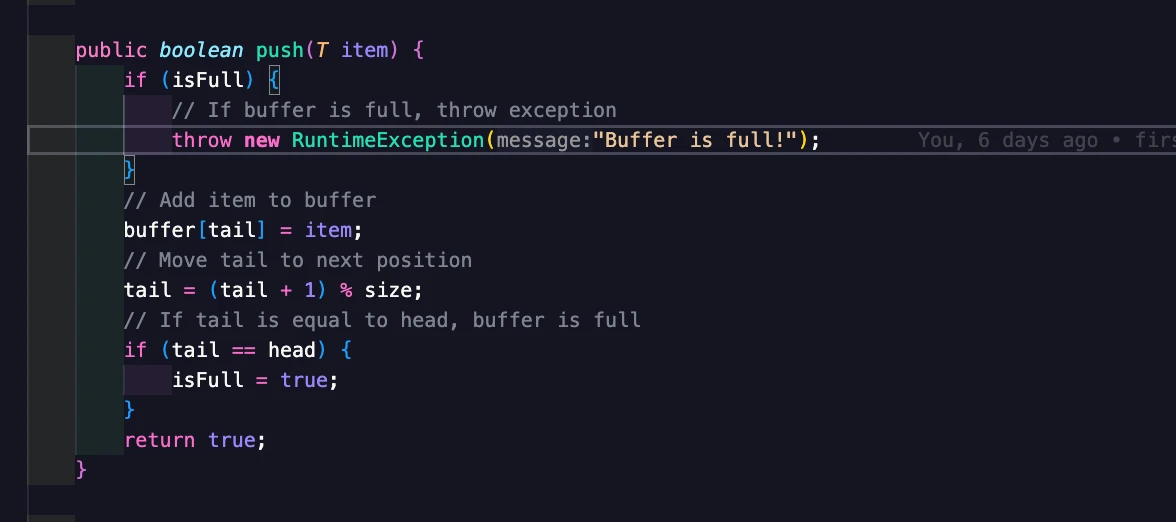
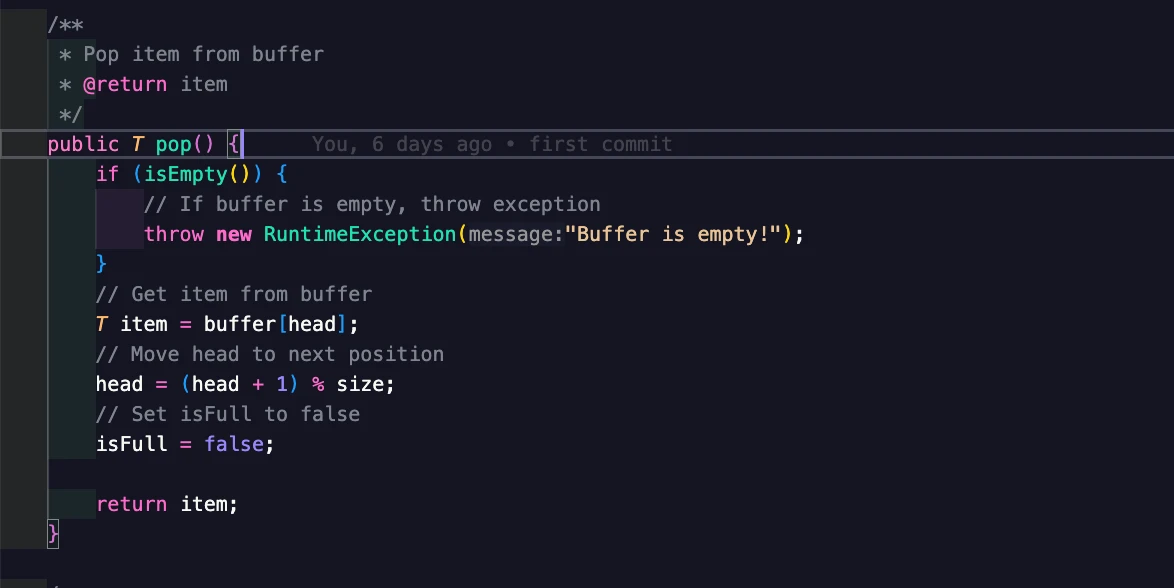
Above are all steps that will help you develop a matching engine using ring buffer. You can follow these steps and enhance the performance of a crypto/foreign/stock exchanges. If want to develop a those exchanges or applicaton that need a matching engine you can contact us, share the project details and get the quotation.
We are always willing to sign an NDA to save your information!